styled-components の特徴と Next.js のプロジェクトへstyled-componentsを導入するまでの手順
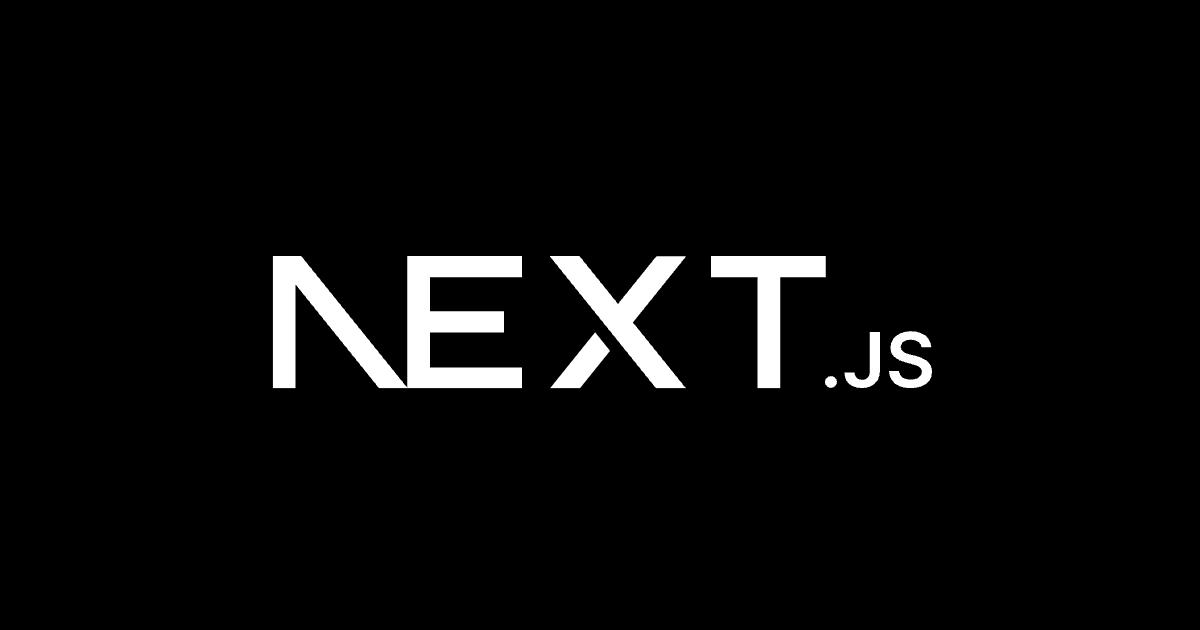
styled-components(スタイルドコンポーネント)
styled-componentsとは?
CSS in JSのライブラリでJSファイルの中にスタイルを記述して利用します。
ReactJSと相性が良くコンポーネント単位でスタイルを記述していくことができます。
公式サイト
styled-componentsを使うメリット
styled-componentsを利用するメリットはたくさんあると感じていますが
私が感じていることを簡潔にまとめています。
コンポーネント管理がしやすい
コンポーネントとスタイルを合わせて管理できるため管理が行いやすいです。
pages/index.tsxconst Title = styled.h1`
color: blue;
font-size: 50px;
`;
const Home = (): ReactElement => {
return <Title>Hello, world!</Title>;
};
このようにコンポーネントのファイルへスタイルを合わせて記述します。
スタイルが閉じられている
コンポーネントのスタイルは同じコンポーネントへしか当たりません。
任意のIDが割り振られ同じコンポーネントのみスタイルが当たるようになあります。
コンパイル後
php-template<style>
.bQcLNl {
color: blue;
font-size: 50px;
}
</style>
<h1 class="pages__Title-sc-16nru3a-0 bQcLNl">Hello, world!</h1>
スタイルの影響を防ぐためにBEPなどの複雑な命名規則を考える必要がありません。
CSSの文法をそのまま利用できる
オブジェクトではなくCSSの文法をそのまま利用できます。
pages/index.tsxconst Title = styled.h1`
color: blue;
font-size: 50px;
`;
それでは早速使っていきます。
環境
- Mac OS Big SUR 11.3.1
- yarn 1.22.10
- Node 14.15.3
- Next.js 10.2.3
- styled-components 5.3
ファイル操作で利用する Unix コマンドについて
基本的なディレクトリ作成やファイル操作は Unix コマンドを利用します。
Unix コマンドについて詳しくはこちらの記事を参考にしてください。
Next.js 環境の構築
前回Next.js
をインストールした環境の状態から変更を加えて行きます。
前回の記事については下記になりますので事前にご確認いただければと思います。
styled-componentsのインストール
styled-componentsのインストール
パッケージのインストール
プロジェクトディレクトリへ移動
前回作成したプロジェクトのディレクトリへ移動します。
terminalcd ~/next-sample
styled-components のインストール
yarn install
コマンドでstyled-components
をインストールします。
terminal$ yarn add styled-components
yarn add v1.22.10
// 中略
✨ Done in 4.67s.
関連パッケージのインストール
yarn install
コマンドでbabel-plugin-styled-components
と@types/styled-components
をインストールします。
terminal$ yarn add -D babel-plugin-styled-components @types/styled-components
yarn add v1.22.10
info No lockfile found.
// 中略
success Saved lockfile.
success Saved 3 new dependencies.
info Direct dependencies
├─ @types/styled-components@5.1.10
└─ babel-plugin-styled-components@1.12.0
info All dependencies
├─ @types/hoist-non-react-statics@3.3.1
├─ @types/styled-components@5.1.10
└─ babel-plugin-styled-components@1.12.0
✨ Done in 4.71s.
@types/styled-components
styled-componentsの型定義ファイルになります。
babel-plugin-styled-components
styled-componentsのデバッグ系の設定を追加したり
SSRで利用できるようにするための設定を拡張するプラグインになります。
インストールしたパッケージの確認
package.json
を開きインストールされたパッケージを確認します。
perl "dependencies": {
"next": "^10.2.3",
"react": "^17.0.2",
"react-dom": "^17.0.2",
"styled-components": "^5.3.0"
},
"devDependencies": {
"@types/node": "^15.12.2",
"@types/react": "^17.0.11",
"@types/styled-components": "^5.1.10",
"babel-plugin-styled-components": "^1.12.0",
"typescript": "^4.3.2"
}
サンプルアプリの変更
前回作成したNext.jsのアプリケーションへ変更を加えていきます。
styled-componentsをNext.jsで使う設定
styled-componentsを利用できるようにするために
pages ディレクトリの直下に_document.tsx
を作成します。
terminal$ vim ~/next-sample/pages/_document.tsx
下記内容を追記します。
_document.tsx
_document.tsximport Document, { DocumentContext } from 'next/document'
import { ServerStyleSheet } from 'styled-components'
export default class MyDocument extends Document {
static async getInitialProps(ctx: DocumentContext) {
const sheet = new ServerStyleSheet()
const originalRenderPage = ctx.renderPage
try {
ctx.renderPage = () =>
originalRenderPage({
enhanceApp: (App) => (props) =>
sheet.collectStyles(<App {...props} />),
})
const initialProps = await Document.getInitialProps(ctx)
return {
...initialProps,
styles: (
<>
{initialProps.styles}
{sheet.getStyleElement()}
</>
),
}
} finally {
sheet.seal()
}
}
}
_document.tsxとは
_document.tsx_はNext.jsで提供されているdocument系の定義をいこなうためのコンポーネントです。ここで定義した内容は通常のpages内のコンポーネントのページへアクセスがあった際にサーバー側のレンダリング時に呼ばれます。
index.tsxの変更
styled-componentsをインポートして
実際に使っていきます。
terminal$ vim ~/next-sample/pages/index.tsx
下記内容を追記します。
index.tsx
index.tsximport React, { ReactElement } from 'react';
import styled from 'styled-components';
const Title = styled.h1`
color: blue;
font-size: 50px;
`;
const Home = (): ReactElement => {
return <Title>Hello, world!</Title>;
};
export default Home;
.babelrcの設定追加
babel
の設定ファイルである.babelrc
を作成し設定内容を追記します。
terminal$ vim ~/next-sample/.babelrc
下記を追記します。
追記する内容
json{
"presets": ["next/babel"],
"plugins": [
[
"styled-components",
{ "ssr": true, "displayName": true }
]
]
}
ssr
サーバーサイドレンダリング時に利用できるようにします。
displayName
コンポーネントが書かれているファイル名をクラス名に出力することができます。
babelとは
JavaScriptのコードを変換するツールです。
次の世代のJavaScriptの標準機能をブラウザのサポートを待たずに使えるようにします。
Next.js アプリの起動
アプリケーションを起動させていきます。
起動コマンドの実行
yarn dev
コマンド実行します。
terminal$ yarn dev
ready - started server on 0.0.0.0:3000, url: http://localhost:3000
// 中略
wait - compiling...
event - compiled successfully
コンソールへsuccessfully
と表示されたらアプリケーション起動は成功です。
ブラウザからアクセス
アプリケーションが起動したのでブラウザからアクセスしてみます。
http:localhost:3000
へアクセスしてみてください。
青い色のHello, world!と表示が確認できます。