TypeScript でよく発生するエラーの原因と解決法まとめ 30 選
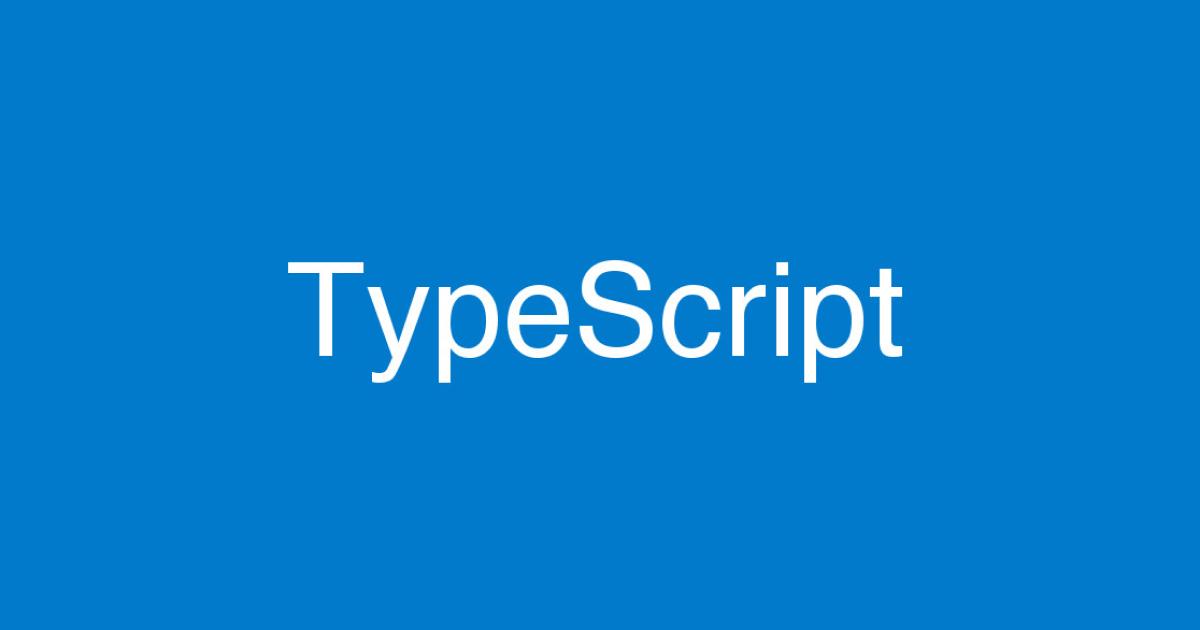
TypeScript を使った開発では、様々なエラーに遭遇します。エラーメッセージを正しく理解し、適切に対処することで開発効率が大きく向上します。この記事では、TypeScript でよく発生する 30 のエラーとその解決法をご紹介します。
TypeScript エラーの基本
エラーは主に型の不一致、構文エラー、設定ミスなどから発生します。これらを理解することで、より効率的なデバッグが可能になるかと思います。
よく発生する TypeScript エラー 30 選
1. TS2322: 型の互換性エラー
エラーメッセージ
pythonTS2322: Type 'string' is not assignable to type 'number'.
このエラーは異なる型同士を代入しようとしたときに発生します。
typescript// エラーコード
let age: number;
age = '30'; // エラー: 文字列を数値型の変数に代入できない
解決策
変数の型に合わせた値を代入するか、適切な型変換を行います。
typescript// 正しいコード
let age: number;
age = 30; // OK
// 型変換を行う場合
let input = '30';
age = parseInt(input); // 文字列を数値に変換
2. TS2339: プロパティやメソッドが存在しないエラー
エラーメッセージ
vbnetTS2339: Property 'toUppercase' does not exist on type 'string'.
このエラーは、存在しないプロパティやメソッドにアクセスしようとしたときに発生します。
typescript// エラーコード
const name = 'TypeScript';
name.toUppercase(); // エラー: toUppercaseというメソッドは存在しない
解決策
正しいメソッド名やプロパティ名を使用します。
typescript// 正しいコード
const name = 'TypeScript';
name.toUpperCase(); // OK: 正しいメソッド名はtoUpperCase
3. TS2531: オブジェクトが null の可能性があるエラー
エラーメッセージ
vbnetTS2531: Object is possibly 'null'.
null の可能性のあるオブジェクトに安全でないアクセスをしたときに発生します。
typescript// エラーコード
const element = document.getElementById('app');
element.innerHTML = '<p>Hello</p>'; // エラー: elementがnullの可能性がある
解決策
条件チェックかオプショナルチェイニングを使用します。
typescript// 解決策1: 条件チェック
const element = document.getElementById('app');
if (element) {
element.innerHTML = '<p>Hello</p>'; // OK
}
// 解決策2: オプショナルチェイニング(TypeScript 3.7以降)
const element = document.getElementById('app');
element?.innerHTML = '<p>Hello</p>'; // OK
4. TS2532: オブジェクトが undefined の可能性があるエラー
エラーメッセージ
vbnetTS2532: Object is possibly 'undefined'.
undefined の可能性がある値を安全でない方法で使用したときに発生します。
typescript// エラーコード
const users = ['Alice', 'Bob'];
const firstUser = users.find((user) =>
user.startsWith('A')
);
console.log(firstUser.length); // エラー: firstUserがundefinedの可能性がある
解決策
条件チェックやオプショナルチェイニング、Non-null assertion operator を使用します。
typescript// 解決策1: 条件チェック
const firstUser = users.find((user) =>
user.startsWith('A')
);
if (firstUser) {
console.log(firstUser.length); // OK
}
// 解決策2: オプショナルチェイニング
console.log(firstUser?.length); // OK: undefinedの場合はundefinedを返す
// 解決策3: Non-null assertion operator(非推奨)
console.log(firstUser!.length); // firstUserが必ずnullでないことを断言
5. TS2345: 関数の引数の数が一致しないエラー
エラーメッセージ
pythonTS2345: Argument of type '...' is not assignable to parameter of type '...'.
関数呼び出し時の引数が、定義された引数の型と一致しないときに発生します。
typescript// エラーコード
function greet(name: string, age: number) {
return `Hello, ${name}. You are ${age} years old.`;
}
greet('John'); // エラー: 引数が足りない
greet('John', '30'); // エラー: 2つ目の引数の型が違う
解決策
関数の定義に合わせて正しい型の引数を渡すか、オプショナルパラメータを使用します。
typescript// 解決策1: 正しい引数を渡す
greet('John', 30); // OK
// 解決策2: オプショナルパラメータを使用する
function greet2(name: string, age?: number) {
if (age) {
return `Hello, ${name}. You are ${age} years old.`;
}
return `Hello, ${name}.`;
}
greet2('John'); // OK: ageは省略可能
6. TS2554: 必須の引数が足りないエラー
エラーメッセージ
javascriptTS2554: Expected X arguments, but got Y.
関数呼び出し時に必要な引数の数が足りないときに発生します。
typescript// エラーコード
function multiply(a: number, b: number): number {
return a * b;
}
multiply(5); // エラー: 引数が1つしかない、2つ必要
解決策
必須の引数をすべて渡すか、デフォルト値を設定します。
typescript// 解決策1: すべての引数を渡す
multiply(5, 2); // OK
// 解決策2: デフォルト値を設定する
function multiplyWithDefault(
a: number,
b: number = 1
): number {
return a * b;
}
multiplyWithDefault(5); // OK: bはデフォルト値の1が使われる
7. TS1005: ';' が見つからないエラー
エラーメッセージ
vbnetTS1005: ';' expected.
文の終わりにセミコロンが必要な場所で不足しているときに発生します。
typescript// エラーコード
let x = 10;
let y = 20; // セミコロンが不足している
解決策
文の終わりにセミコロンを追加するか、tsconfig.json で"semicolon": false
を設定します。
typescript// 解決策: セミコロンを追加
let x = 10;
let y = 20;
8. TS2307: モジュールが見つからないエラー
エラーメッセージ
luaTS2307: Cannot find module 'module-name' or its corresponding type declarations.
インポートしようとしているモジュールが見つからないときに発生します。
typescript// エラーコード
import { Button } from 'my-ui-library'; // エラー: モジュールが見つからない
解決策
モジュールをインストールするか、パスを修正します。
bash# 解決策1: モジュールをインストール
yarn add my-ui-library
# 解決策2: 型定義をインストール
yarn add -D @types/my-ui-library
または相対パスが間違っている場合は修正します:
typescript// 間違ったパス
import { Button } from './components/button';
// 正しいパス
import { Button } from './components/Button'; // 大文字小文字も重要
9. TS2339: インターフェースで定義されていないプロパティへのアクセス
エラーメッセージ
vbnetTS2339: Property 'propertyName' does not exist on type 'InterfaceName'.
インターフェースで定義されていないプロパティにアクセスしようとすると発生します。
typescript// エラーコード
interface User {
name: string;
age: number;
}
const user: User = { name: 'Alice', age: 30 };
console.log(user.email); // エラー: emailプロパティはUserインターフェースにない
解決策
インターフェースを拡張するか、存在するプロパティのみを使用します。
typescript// 解決策1: インターフェースを修正
interface User {
name: string;
age: number;
email?: string; // オプショナルプロパティとして追加
}
// 解決策2: 型アサーションを使用(非推奨)
console.log((user as any).email); // 型チェックをバイパスする
10. TS2366: オブジェクトリテラルでプロパティが重複しているエラー
エラーメッセージ
vbnetTS2366: Object literal may only specify known properties.
オブジェクトリテラルで同じプロパティが複数回定義されているときに発生します。
typescript// エラーコード
const person = {
name: 'John',
age: 30,
name: 'Doe', // エラー: nameプロパティが重複している
};
解決策
重複したプロパティを削除するか、名前を変更します。
typescript// 正しいコード
const person = {
name: 'John Doe', // 名前を一つにまとめる
age: 30,
};
// または
const person = {
firstName: 'John',
lastName: 'Doe',
age: 30,
};
11. TS2740: 型のユニオンでプロパティが足りないエラー
エラーメッセージ
pythonTS2740: Type '{ ... }' is missing the following properties from type '...': ...
ユニオン型において、必要なプロパティが不足しているときに発生します。
typescript// エラーコード
type Shape = Circle | Rectangle;
interface Circle {
kind: 'circle';
radius: number;
}
interface Rectangle {
kind: 'rectangle';
width: number;
height: number;
}
// エラー: kindプロパティがない
const shape: Shape = {
radius: 5,
};
解決策
必要なプロパティをすべて指定します。
typescript// 正しいコード
const circle: Shape = {
kind: 'circle',
radius: 5,
}; // OK
const rectangle: Shape = {
kind: 'rectangle',
width: 10,
height: 20,
}; // OK
12. TS2542: インデックスシグネチャのエラー
エラーメッセージ
bashTS2542: Index signature in type '...' only permits reading.
読み取り専用のインデックスシグネチャに値を代入しようとすると発生します。
typescript// エラーコード
interface ReadOnlyArray {
readonly [index: number]: string;
}
const arr: ReadOnlyArray = ['a', 'b', 'c'];
arr[0] = 'x'; // エラー: 読み取り専用の配列に代入できない
解決策
読み取り専用の型を変更するか、新しいオブジェクトを作成します。
typescript// 解決策1: 読み取り専用でない型を使用
interface WritableArray {
[index: number]: string;
}
const arr: WritableArray = ['a', 'b', 'c'];
arr[0] = 'x'; // OK
// 解決策2: 新しい配列を作成
const newArr = ['x', ...arr.slice(1)];
13. TS2352: 型が過剰なプロパティを持つエラー
エラーメッセージ
pythonTS2352: Conversion of type '...' to type '...' may be a mistake because neither type sufficiently overlaps with the other.
オブジェクトに余分なプロパティがあるときに発生します。
typescript// エラーコード
interface Person {
name: string;
age: number;
}
const data = {
name: 'Alice',
age: 30,
email: 'alice@example.com',
};
const person: Person = data; // Typescriptのデフォルト設定では問題ない
// 厳格なチェックが有効な場合のエラー例:
function processPerson(p: Person) {
console.log(p.name, p.age);
}
// エラー: オブジェクトリテラルを直接渡すと過剰プロパティチェックが適用される
processPerson({
name: 'Bob',
age: 25,
email: 'bob@example.com', // 過剰なプロパティ
});
解決策
インデックスシグネチャを追加するか、型アサーションを使用します。
typescript// 解決策1: インターフェースを拡張
interface Person {
name: string;
age: number;
[key: string]: any; // 任意の追加プロパティを許可
}
// 解決策2: 型アサーションを使用
processPerson({
name: 'Bob',
age: 25,
email: 'bob@example.com',
} as Person);
// 解決策3: 変数に代入してから渡す
const bob = {
name: 'Bob',
age: 25,
email: 'bob@example.com',
};
processPerson(bob); // OK: 変数からの代入では過剰プロパティチェックが緩和される
14. TS2578: 列挙型が文字列値を持つエラー
エラーメッセージ
vbnetTS2578: Enum member must have initializer.
値を持たない列挙型のメンバーがある場合に発生します。
typescript// エラーコード
enum Direction {
Up, // 0に自動初期化
Down, // 1に自動初期化
Left = 'LEFT', // 文字列値
Right, // エラー: 前のメンバーが文字列なので初期化子が必要
}
解決策
すべての列挙型メンバーに明示的な値を設定します。
typescript// 正しいコード
enum Direction {
Up = 0,
Down = 1,
Left = 'LEFT',
Right = 'RIGHT',
}
// または数値のみ、または文字列のみに統一
enum NumericDirection {
Up = 0,
Down = 1,
Left = 2,
Right = 3,
}
enum StringDirection {
Up = 'UP',
Down = 'DOWN',
Left = 'LEFT',
Right = 'RIGHT',
}
15. TS2538: readonly プロパティの変更エラー
エラーメッセージ
pythonTS2538: Type '...' cannot be used as an index type.
readonly プロパティを変更しようとすると発生します。
typescript// エラーコード
interface Config {
readonly apiUrl: string;
timeout: number;
}
const config: Config = {
apiUrl: 'https://api.example.com',
timeout: 3000,
};
config.apiUrl = 'https://new-api.example.com'; // エラー: readonlyプロパティは変更できない
解決策
readonly プロパティを変更するには、新しいオブジェクトを作成します。
typescript// 解決策: 新しいオブジェクトを作成
const newConfig: Config = {
...config,
apiUrl: 'https://new-api.example.com',
};
// または特定のケースではこれも可能
const mutableConfig = config as {
apiUrl: string;
timeout: number;
};
mutableConfig.apiUrl = 'https://new-api.example.com';
16. TS2550: 型定義で初期化されていないプロパティのエラー
エラーメッセージ
vbnetTS2550: Property '...' does not exist on type '...'. Did you mean '...'?
クラスで定義したプロパティが初期化されていないときに発生します。
typescript// エラーコード
class Person {
name: string; // プロパティが初期化されていない
age: number;
constructor() {
// nameが初期化されていない
this.age = 0;
}
}
解決策
コンストラクタでプロパティを初期化するか、初期値を設定します。
typescript// 解決策1: コンストラクタで初期化
class Person {
name: string;
age: number;
constructor(name: string) {
this.name = name;
this.age = 0;
}
}
// 解決策2: 初期値を設定
class Person {
name: string = '';
age: number = 0;
constructor(name?: string) {
if (name) {
this.name = name;
}
}
}
// 解決策3: strictPropertyInitializationを無効にする(非推奨)
class Person {
name!: string; // !演算子で初期化を強制的にバイパス
age: number = 0;
}
17. TS2698: スプレッド演算子で Unknown 型が使われるエラー
エラーメッセージ
csharpTS2698: Spread operator for type '...' is not supported.
型情報が不明な値に対してスプレッド演算子を使用すると発生します。
typescript// エラーコード
function merge(obj1: unknown, obj2: unknown) {
return { ...obj1, ...obj2 }; // エラー: unknownにスプレッド演算子を使えない
}
解決策
型アサーションを使用するか、型ガードを追加します。
typescript// 解決策1: 型アサーション
function merge(obj1: unknown, obj2: unknown) {
return { ...(obj1 as object), ...(obj2 as object) };
}
// 解決策2: 型ガード
function merge(obj1: unknown, obj2: unknown) {
if (typeof obj1 !== 'object' || obj1 === null) {
obj1 = {};
}
if (typeof obj2 !== 'object' || obj2 === null) {
obj2 = {};
}
return { ...obj1, ...obj2 };
}
// 解決策3: ジェネリック型を使用(推奨)
function merge<T extends object, U extends object>(
obj1: T,
obj2: U
) {
return { ...obj1, ...obj2 };
}
18. TS2416: クラスプロパティの修飾子の競合エラー
エラーメッセージ
pythonTS2416: Property '...' in type '...' is not assignable to the same property in base type '...'.
サブクラスでプロパティの修飾子(readonly、private 等)が基底クラスと競合すると発生します。
typescript// エラーコード
class Base {
public name: string = 'base';
}
class Derived extends Base {
private name: string = 'derived'; // エラー: 基底クラスではpublicだが派生クラスではprivate
}
解決策
基底クラスと同じアクセス修飾子を使用します。
typescript// 解決策: 修飾子を合わせる
class Base {
public name: string = 'base';
}
class Derived extends Base {
public name: string = 'derived'; // OK: 両方ともpublic
}
19. TS2564: プロパティは undefined を許可しないが初期化されていないエラー
エラーメッセージ
vbnetTS2564: Property '...' has no initializer and is not definitely assigned in the constructor.
プロパティが初期化されておらず、undefined も許可していない場合に発生します。
typescript// エラーコード
class User {
name: string; // 初期化されていない
constructor() {
// nameが初期化されていない
}
}
解決策
プロパティを初期化するか、型に undefined を追加します。
typescript// 解決策1: 初期化
class User {
name: string = ''; // 初期値を設定
constructor(name?: string) {
if (name) {
this.name = name;
}
}
}
// 解決策2: undefinedを許可
class User {
name: string | undefined;
}
// 解決策3: 確定代入アサーション(非推奨)
class User {
name!: string; // !演算子で「必ず初期化される」と断言
initialize(name: string) {
this.name = name;
}
}
20. TS2349: 型の互換性がないメソッドの実装エラー
エラーメッセージ
pythonTS2349: This expression is not callable.
関数型に互換性がない場合に発生します。
typescript// エラーコード
type Callback = (x: number) => void;
const myFunction: Callback = (x: string) => {
// エラー: 引数の型が不一致
console.log(x.toUpperCase());
};
解決策
型定義に合わせて関数を実装します。
typescript// 解決策: 正しい型で実装
const myFunction: Callback = (x: number) => {
console.log(x.toString());
};
21. TS7031: バインディングエレメントがないエラー
エラーメッセージ
bashTS7031: Binding element '...' implicitly has an 'any' type.
関数の引数で分割代入を使用し、型を指定していない場合に発生します。
typescript// エラーコード
function printUserInfo({ name, age }) {
// エラー: 暗黙的にanyになっている
console.log(`${name}, ${age}`);
}
解決策
引数オブジェクトの型を明示的に指定します。
typescript// 解決策1: インラインで型を指定
function printUserInfo({
name,
age,
}: {
name: string;
age: number;
}) {
console.log(`${name}, ${age}`);
}
// 解決策2: インターフェースを使用
interface UserInfo {
name: string;
age: number;
}
function printUserInfo({ name, age }: UserInfo) {
console.log(`${name}, ${age}`);
}
22. TS2556: タイプチェックなしにオブジェクトにアクセスするエラー
エラーメッセージ
pythonTS2556: A spread argument must either have a tuple type or be passed to a rest parameter.
スプレッド構文を使用してタプル型でない配列を展開しようとすると発生します。
typescript// エラーコード
function concat(arr1: unknown, arr2: unknown) {
return [...arr1, ...arr2]; // エラー: arr1とarr2がタプル型または配列型でない
}
解決策
適切な型チェックを追加します。
typescript// 解決策1: ジェネリック型を使用
function concat<T>(arr1: T[], arr2: T[]): T[] {
return [...arr1, ...arr2];
}
// 解決策2: 型チェックを追加
function concat(arr1: unknown, arr2: unknown) {
if (Array.isArray(arr1) && Array.isArray(arr2)) {
return [...arr1, ...arr2];
}
throw new Error('Both arguments must be arrays');
}
23. TS2322: 異なるタイプのオブジェクトをマージするエラー
エラーメッセージ
pythonTS2322: Type '{ ... }' is not assignable to type '...'.
異なる型を持つオブジェクトを統合しようとすると発生します。
typescript// エラーコード
interface User {
id: number;
name: string;
}
const partialUser = { name: 'John' };
const user: User = partialUser; // エラー: idプロパティがない
解決策
必要なプロパティをすべて指定するか、Partial型を使用します。
typescript// 解決策1: すべてのプロパティを指定
const user: User = {
id: 1,
name: 'John',
};
// 解決策2: Partial型を使用
const partialUser: Partial<User> = { name: 'John' };
// 解決策3: 型アサーションを使用(不足プロパティの責任を開発者が負う)
const user: User = { name: 'John' } as User;
// 解決策4: スプレッド構文でオブジェクトをマージ
const defaultUser: User = { id: 0, name: '' };
const user: User = { ...defaultUser, ...partialUser };
24. TS2589: 型推論の制限エラー
エラーメッセージ
pythonTS2589: Type instantiation is excessively deep and possibly infinite.
循環参照や複雑な型定義で型推論のスタックオーバーフローが発生すると出ます。
typescript// エラーコード
type RecursiveType<T> = {
value: T;
next: RecursiveType<T>; // エラー: 無限に再帰する型
};
const list: RecursiveType<number> = {
value: 1,
next: {
/* ... */
}, // 無限に続く構造
};
解決策
再帰型には null または undefined の場合を追加します。
typescript// 解決策: 終端条件を追加
type RecursiveType<T> = {
value: T;
next: RecursiveType<T> | null; // nullで終端できるようにする
};
const list: RecursiveType<number> = {
value: 1,
next: {
value: 2,
next: null, // ここで終端
},
};
25. TS2739: 型アサーションのエラー
エラーメッセージ
pythonTS2739: Type '...' is missing the following properties from type '...': ...
互換性のない型へのアサーションを行うと発生します。
typescript// エラーコード
interface User {
id: number;
name: string;
email: string;
}
const partialData = { id: 1 };
const user = partialData as User; // エラー: nameとemailプロパティがない
解決策
互換性のある型を使用するか、二重アサーションを使用します。
typescript// 解決策1: 必要なプロパティをすべて指定
const user = {
id: 1,
name: '',
email: '',
} as User;
// 解決策2: Partial<T>を使用
const partialUser = partialData as Partial<User>;
// 解決策3: 二重アサーションを使用(非推奨)
const user = partialData as unknown as User;
26. TS1208: tsconfig.json のエラー
エラーメッセージ
vbnetTS1208: 'tsconfig.json' cannot be opened: ...
tsconfig.json が見つからないか、JSON として無効な場合に発生します。
typescript// エラーではなく設定ファイルの問題
// 無効なtsconfig.jsonの例
{
"compilerOptions": {
"target": "es5"
"module": "commonjs" // カンマが不足している
}
}
解決策
tsconfig.json の構文を修正します。
json// 正しいtsconfig.json
{
"compilerOptions": {
"target": "es5",
"module": "commonjs"
}
}
27. TS2749: JSX の型定義エラー
エラーメッセージ
pythonTS2749: 'Component' refers to a value, but is being used as a type here.
JSX コンポーネントを型として使用しようとすると発生します。
typescript// エラーコード
import { Button } from 'my-ui-library';
// エラー: Buttonは値として扱われるべき
function renderButton(props: Button) {
return <Button {...props} />;
}
解決策
コンポーネントの Prop の型を使用します。
typescript// 解決策
import { Button, ButtonProps } from 'my-ui-library';
// ButtonPropsを型として使用
function renderButton(props: ButtonProps) {
return <Button {...props} />;
}
// React.ComponentPropsを使用する方法もある
import React from 'react';
import { Button } from 'my-ui-library';
function renderButton(
props: React.ComponentProps<typeof Button>
) {
return <Button {...props} />;
}
28. TS2786: 'this'の型が失われるエラー
エラーメッセージ
pythonTS2786: 'this' refers to a value, but is being used as a type here.
コールバック関数内で this の型が失われたときに発生します。
typescript// エラーコード
class Counter {
count = 0;
increment() {
this.count++;
}
start() {
// エラー: setIntervalのコールバック内でthisが失われる
setInterval(function () {
this.increment();
}, 1000);
}
}
解決策
アロー関数を使用するか、bind メソッドを使用します。
typescript// 解決策1: アロー関数を使用
class Counter {
count = 0;
increment() {
this.count++;
}
start() {
// OK: アロー関数はthisをレキシカルスコープから取得
setInterval(() => {
this.increment();
}, 1000);
}
}
// 解決策2: bindメソッドを使用
class Counter {
count = 0;
increment() {
this.count++;
}
start() {
setInterval(
function () {
this.increment();
}.bind(this),
1000
);
}
}
29. TS1337: レガシーモジュールインポートのエラー
エラーメッセージ
vbnetTS1337: An import path cannot end with a '.ts' extension.
.ts ファイルを直接インポートしようとしたときに発生します。
typescript// エラーコード
import { someFunction } from './utils.ts'; // エラー: .ts拡張子は使用できない
解決策
拡張子を省略します。
typescript// 正しいコード
import { someFunction } from './utils'; // OK
30. TS7016: 型定義のないモジュールのエラー
エラーメッセージ
arduinoTS7016: Could not find a declaration file for module '...'.
型定義が不足しているモジュールをインポートしたときに発生します。
typescript// エラーコード
import * as someLibrary from 'some-library'; // エラー: 型定義が見つからない
解決策
型定義パッケージをインストールするか、declare ステートメントを追加します。
bash# 解決策1: 型定義パッケージをインストール
yarn add -D @types/some-library
typescript// 解決策2: モジュール宣言を追加
// src/types/some-library.d.ts などのファイルを作成
declare module 'some-library' {
export function someFunction(): void;
// 他の必要な型定義
}
TypeScript の型システムを理解するための重要なポイント
TypeScript のエラーに効率的に対処するには、型システムの基本概念を理解することが重要です。型の互換性、共変性と反変性、型推論のメカニズムなどの知識があると、エラーメッセージの理解と解決が容易になります。
TypeScript の設定ファイル(tsconfig.json)のオプションも理解しておくと便利です。特にstrict
モードやnoImplicitAny
などの設定は、コードの品質を高める一方で、多くのエラーを表示することがあります。
効率的なデバッグのためのヒント
-
型情報を活用する: VSCode などのエディタでは、変数やプロパティにカーソルを合わせると型情報が表示されます。この情報を活用してエラーの原因を特定しましょう。
-
エラーメッセージを注意深く読む: TypeScript のエラーメッセージは詳細な情報を提供しています。エラーメッセージの最初の部分だけでなく、詳細部分もしっかり読むことでヒントが得られます。
-
型ガードを活用する:
typeof
、instanceof
、カスタム型ガード関数を使用することで、ランタイムでの型チェックが可能になります。 -
段階的な型付け: 大規模なコードベースでは、すべてを一度に型付けするのではなく、段階的に重要な部分から型付けを進めましょう。
any
型から始めて徐々に具体的な型に変更する方法も効果的です。
まとめ
TypeScript のエラーは最初は複雑に見えますが、パターンを理解するとデバッグが容易になります。この記事で紹介したエラーと解決策を参考に、TypeScript の型システムをより深く理解し、効率的な開発を進めていただければ幸いです。
型エラーは「敵」ではなく、コードの品質を高める「味方」です。エラーメッセージを的確に理解し、適切に対処することで、より堅牢で保守性の高いコードを書くことができます。
関連リンク
- review
今の自分に満足していますか?『持たざる者の逆襲 まだ何者でもない君へ』溝口勇児
- review
ついに語られた業界の裏側!『フジテレビの正体』堀江貴文が描くテレビ局の本当の姿
- review
愛する勇気を持てば人生が変わる!『幸せになる勇気』岸見一郎・古賀史健のアドラー実践編で真の幸福を手に入れる
- review
週末を変えれば年収も変わる!『世界の一流は「休日」に何をしているのか』越川慎司の一流週末メソッド
- review
新しい自分に会いに行こう!『自分の変え方』村岡大樹の認知科学コーチングで人生リセット
- review
科学革命から AI 時代へ!『サピエンス全史 下巻』ユヴァル・ノア・ハラリが予見する人類の未来