クラス名が長すぎる?Tailwind CSSで読みやすさを保つ設計術
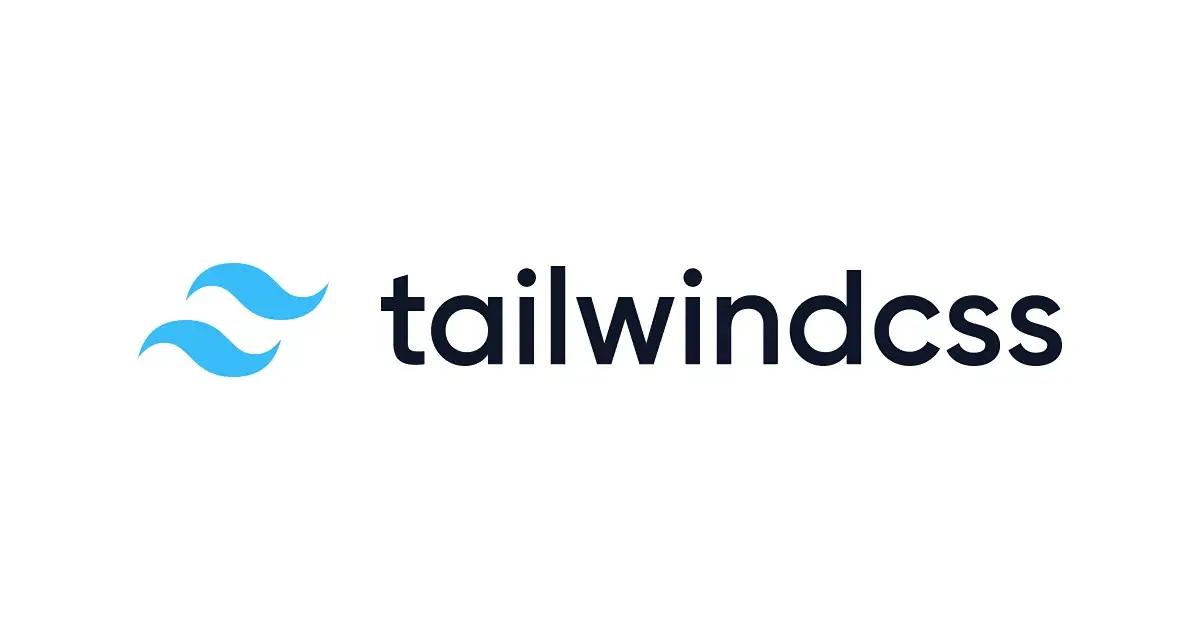
html<div class="flex items-center justify-between p-4 bg-blue-50 rounded-lg shadow-sm hover:shadow-md transition-shadow duration-300 sm:p-6 md:p-8 lg:p-10">
こんなに長いクラス名、見た瞬間に頭が痛くなりませんか?Tailwind CSS を使っていると、このような長大なクラス名の連なりに出会うことは珍しくありません。ユーティリティファーストの強力さと引き換えに、私たちは読みにくい HTML と格闘することになるのでしょうか?
今回は、Tailwind CSS の最大の弱点とも言われる「長いクラス名」の問題に焦点を当て、実践的な解決策を詳しく解説していきます。コードの可読性を保ちながら、Tailwind の恩恵を最大限に活かす方法を見ていきましょう。
背景(ユーティリティファーストの読みやすさへの影響)
Tailwind の成功とその代償
Tailwind CSS はここ数年で爆発的な人気を獲得しました。その人気の理由は明らかです:
- HTML 内で直接スタイリングできる即時性
- クラスを組み合わせる柔軟性
- 一貫したデザインシステムの適用しやすさ
しかし、この「ユーティリティファースト」という強みは、皮肉なことに Tailwind の最大の批判点にもなっています。
なぜクラス名が肥大化するのか
Tailwind のクラス名が長くなる主な理由は、以下のようなパターンの組み合わせにあります:
- 基本的なスタイル適用 (
bg-blue-500
,text-lg
) - レスポンシブ対応 (
sm:flex
,md:grid
,lg:hidden
) - 状態変化の指定 (
hover:bg-blue-600
,focus:outline-none
) - ダークモード対応 (
dark:bg-slate-800
,dark:text-white
) - アニメーションや変化 (
transition-all
,duration-300
)
これらが組み合わさると、下記のようなコードが生まれます:
html<button
class="px-4 py-2 text-white bg-blue-500 rounded-lg shadow-md hover:bg-blue-600 focus:outline-none focus:ring-2 focus:ring-blue-500 focus:ring-opacity-50 transition-colors duration-300 dark:bg-blue-700 dark:hover:bg-blue-800 md:px-6 md:py-3"
>
送信する
</button>
このアプローチによって得られる開発速度と柔軟性は素晴らしいものですが、HTML の可読性という観点では明らかに問題があります。
課題(HTML 可読性の低下とコードの管理難)
Tailwind の長大なクラス名は、以下のような具体的な問題を引き起こします:
可読性の低下
html<div
class="flex flex-col items-center justify-between p-4 bg-white rounded-lg shadow-lg dark:bg-gray-800 dark:text-white sm:flex-row sm:items-start md:p-6 lg:p-8"
>
<h2
class="mb-4 text-xl font-bold text-gray-900 dark:text-white sm:mb-0"
>
タイトル
</h2>
<p class="text-gray-600 dark:text-gray-300">説明文</p>
</div>
このようなコードを見た時:
- どのクラスがどの視覚的効果を担当しているのか把握しづらい
- HTML 要素の階層構造が見えづらい
- コードの全体像を素早く理解することが困難
管理の難しさ
長いクラス名は、以下のような管理上の問題も引き起こします:
- 重複の発生:同じクラスの組み合わせが複数の場所で使われる
- 一貫性の欠如:似た UI でもわずかに異なるクラス設定になりがち
- 更新の複雑性:デザイン変更時に多くの場所を修正する必要がある
コード編集の非効率性
html<div
class="grid grid-cols-1 gap-4 sm:grid-cols-2 md:grid-cols-3 lg:grid-cols-4"
>
<!-- 各アイテム -->
<div
class="p-4 bg-white rounded-lg shadow dark:bg-gray-800"
>
...
</div>
<div
class="p-4 bg-white rounded-lg shadow dark:bg-gray-800"
>
...
</div>
<div
class="p-4 bg-white rounded-lg shadow dark:bg-gray-800"
>
...
</div>
<!-- 以下、同じパターンが続く -->
</div>
繰り返し同じクラス名を使うことは:
- タイピング量の増加
- コピー&ペーストによるミス発生リスク
- 後からの一括変更の難しさ
これらの課題を解決するには、いくつかの効果的なアプローチがあります。
解決策(@apply ディレクティブとコンポーネント抽出)
Tailwind の長いクラス名問題を解決するために、主に以下の戦略が効果的です:
1. @apply ディレクティブの活用
最も直接的な解決策の一つが、Tailwind の@apply
ディレクティブです。これを使うと、複数のユーティリティクラスをカスタム CSS クラスにまとめることができます。
css/* styles.css */
.btn-primary {
@apply px-4 py-2 text-white bg-blue-500 rounded-lg shadow-md hover:bg-blue-600 focus:outline-none focus:ring-2 focus:ring-blue-500 focus:ring-opacity-50 transition-colors duration-300;
}
.card {
@apply p-4 bg-white rounded-lg shadow dark:bg-gray-800;
}
これで、HTML は以下のようにすっきりします:
html<button
class="btn-primary md:px-6 md:py-3 dark:bg-blue-700 dark:hover:bg-blue-800"
>
送信する
</button>
<div class="card">コンテンツ</div>
@apply のベストプラクティス
- 意味のある命名 - BEM などの命名規則に従う
- 最小限の抽象化 - 頻繁に再利用するパターンのみ抽出する
- 変更可能性を考慮 - レスポンシブや状態変化のクラスは分離を検討
2. コンポーネント抽出
モダンなフレームワーク(React、Vue、Svelte など)を使用している場合、コンポーネントに分割することでコードの可読性と再利用性を高めることができます。
React の例
jsx// Button.jsx
function Button({ children, className = '' }) {
return (
<button
className={`px-4 py-2 text-white bg-blue-500 rounded-lg shadow-md
hover:bg-blue-600 focus:outline-none focus:ring-2
focus:ring-blue-500 focus:ring-opacity-50
transition-colors duration-300 ${className}`}
>
{children}
</button>
);
}
// 使用例
<Button className='md:px-6 md:py-3'>送信する</Button>;
Vue の例
vue<!-- Button.vue -->
<template>
<button :class="['btn-base', $attrs.class]">
<slot></slot>
</button>
</template>
<script setup>
// コンポーネントのロジック
</script>
<style scoped>
.btn-base {
@apply px-4 py-2 text-white bg-blue-500 rounded-lg shadow-md
hover:bg-blue-600 focus:outline-none focus:ring-2
focus:ring-blue-500 focus:ring-opacity-50
transition-colors duration-300;
}
</style>
<!-- 使用例 -->
<Button class="md:px-6 md:py-3">送信する</Button>
3. Tailwind の設定ファイルのカスタマイズ
繰り返し使われるデザインパターンは、Tailwind の設定ファイルで定義することも効果的です:
js// tailwind.config.js
module.exports = {
theme: {
extend: {
// カスタムカラーの定義
colors: {
brand: {
light: '#3482F6',
DEFAULT: '#2563EB',
dark: '#1D4ED8',
},
},
// カスタムボーダーラジウスの定義
borderRadius: {
card: '0.5rem',
},
// カスタム影の定義
boxShadow: {
card: '0 2px 4px rgba(0,0,0,0.1)',
},
},
},
// 以下、プラグインなど
};
これにより、bg-brand
、rounded-card
、shadow-card
などのカスタムユーティリティクラスが使えるようになります。
具体例(リファクタリング前後のコード比較)
実際にコードをリファクタリングする例を見ていきましょう。
リファクタリング前のコード
html<!-- 商品カードのコンポーネント -->
<div
class="flex flex-col overflow-hidden bg-white rounded-lg shadow-lg dark:bg-gray-800"
>
<img
class="object-cover w-full h-48"
src="product-image.jpg"
alt="商品画像"
/>
<div class="p-4 md:p-6">
<h3
class="text-xl font-semibold text-gray-800 dark:text-white"
>
商品名
</h3>
<p class="mt-2 text-gray-600 dark:text-gray-300">
商品の説明文がここに入ります。
</p>
<div class="flex items-center justify-between mt-4">
<span
class="text-xl font-bold text-gray-900 dark:text-white"
>¥9,800</span
>
<button
class="px-4 py-2 font-bold text-white bg-blue-500 rounded hover:bg-blue-600 focus:outline-none focus:ring-2 focus:ring-blue-500 focus:ring-opacity-50"
>
カートに追加
</button>
</div>
</div>
</div>
リファクタリング方法 1: @apply を使ったアプローチ
css/* styles.css */
.product-card {
@apply flex flex-col overflow-hidden bg-white rounded-lg shadow-lg dark:bg-gray-800;
}
.product-image {
@apply object-cover w-full h-48;
}
.product-content {
@apply p-4 md:p-6;
}
.product-title {
@apply text-xl font-semibold text-gray-800 dark:text-white;
}
.product-description {
@apply mt-2 text-gray-600 dark:text-gray-300;
}
.product-footer {
@apply flex items-center justify-between mt-4;
}
.product-price {
@apply text-xl font-bold text-gray-900 dark:text-white;
}
.btn-add-to-cart {
@apply px-4 py-2 font-bold text-white bg-blue-500 rounded hover:bg-blue-600 focus:outline-none focus:ring-2 focus:ring-blue-500 focus:ring-opacity-50;
}
html<!-- リファクタリング後 -->
<div class="product-card">
<img
class="product-image"
src="product-image.jpg"
alt="商品画像"
/>
<div class="product-content">
<h3 class="product-title">商品名</h3>
<p class="product-description">
商品の説明文がここに入ります。
</p>
<div class="product-footer">
<span class="product-price">¥9,800</span>
<button class="btn-add-to-cart">カートに追加</button>
</div>
</div>
</div>
リファクタリング方法 2: React コンポーネント化
jsx// ProductCard.jsx
function ProductCard({ product }) {
return (
<div className='flex flex-col overflow-hidden bg-white rounded-lg shadow-lg dark:bg-gray-800'>
<img
className='object-cover w-full h-48'
src={product.image}
alt={product.name}
/>
<div className='p-4 md:p-6'>
<h3 className='text-xl font-semibold text-gray-800 dark:text-white'>
{product.name}
</h3>
<p className='mt-2 text-gray-600 dark:text-gray-300'>
{product.description}
</p>
<div className='flex items-center justify-between mt-4'>
<span className='text-xl font-bold text-gray-900 dark:text-white'>
¥{product.price}
</span>
<button
className='px-4 py-2 font-bold text-white bg-blue-500 rounded hover:bg-blue-600 focus:outline-none focus:ring-2 focus:ring-blue-500 focus:ring-opacity-50'
onClick={() => product.addToCart()}
>
カートに追加
</button>
</div>
</div>
</div>
);
}
// 使用例
function ProductList({ products }) {
return (
<div className='grid grid-cols-1 gap-6 md:grid-cols-2 lg:grid-cols-3'>
{products.map((product) => (
<ProductCard key={product.id} product={product} />
))}
</div>
);
}
リファクタリング方法 3: コンポーネントと@apply の組み合わせ
最も効果的なのは、コンポーネント化と@apply
を組み合わせる方法です:
jsx// ProductCard.jsx
import './ProductCard.css';
function ProductCard({ product }) {
return (
<div className='product-card'>
<img
className='product-image'
src={product.image}
alt={product.name}
/>
<div className='product-content'>
<h3 className='product-title'>{product.name}</h3>
<p className='product-description'>
{product.description}
</p>
<div className='product-footer'>
<span className='product-price'>
¥{product.price}
</span>
<button
className='btn-add-to-cart'
onClick={() => product.addToCart()}
>
カートに追加
</button>
</div>
</div>
</div>
);
}
css/* ProductCard.css */
.product-card {
@apply flex flex-col overflow-hidden bg-white rounded-lg shadow-lg dark:bg-gray-800;
}
/* その他のスタイルも同様に... */
リファクタリングのバランス
完全に Tailwind のクラス名をカスタムクラスに置き換えるのではなく、以下のバランスを考慮するのがおすすめです:
-
よく使うパターンだけ抽象化する
- カード、ボタン、フォーム要素など汎用的な UI コンポーネント
-
一度限りのスタイルはインラインで
- 特定の場所でだけ使う調整用のマージンやパディングなど
-
レスポンシブや状態変化は柔軟に
md:
,hover:
,dark:
などの修飾子はしばしばインラインで書くのが合理的
実際の使用例
html<!-- バランスの取れたアプローチ -->
<div class="product-card md:flex-row">
<img
class="product-image md:w-1/3 md:h-auto"
src="product-image.jpg"
alt="商品画像"
/>
<div class="product-content">
<!-- 内容 -->
</div>
</div>
まとめ
Tailwind CSS は開発速度と柔軟性の面で大きなメリットをもたらしますが、長いクラス名による可読性の低下は確かに課題です。しかし、以下の戦略を適切に組み合わせることでこの問題は大幅に緩和できます:
-
@apply ディレクティブの活用
- 繰り返し使われるクラスの組み合わせを抽象化
- 意味のある名前を付けてコードの意図を明確に
-
コンポーネント化
- UI のパーツごとに再利用可能なコンポーネントを作成
- フレームワークの機能を活用してプロップスやスロットで柔軟性を持たせる
-
バランスの取れたアプローチ
- 頻繁に使用するパターンのみ抽象化
- 一度限りのスタイルや変更の多い部分はインラインのまま
これらの方法を状況に応じて使い分けることで、Tailwind の生産性を維持しながら、コードの可読性と保守性を高めることができます。
最も重要なのは、チーム内で一貫したルールを決めて守ることです。何をコンポーネント化し、何を@apply で抽象化するのか、どのような命名規則を使うのかなどのガイドラインを作成することで、長期的に管理しやすいコードベースを構築できるでしょう。
関連リンク
- review
チーム開発が劇的に変わった!『リーダブルコード』Dustin Boswell & Trevor Foucher
- review
アジャイル初心者でも大丈夫!『アジャイルサムライ − 達人開発者への道』Jonathan Rasmusson
- review
人生が作品になる!『自分の中に毒を持て』岡本太郎
- review
体調不良の 99%が解決!『眠れなくなるほど面白い 図解 自律神経の話』小林弘幸著で学ぶ、現代人必須の自律神経コントロール術と人生を変える健康革命
- review
衝撃の事実!『睡眠こそ最強の解決策である』マシュー・ウォーカー著が明かす、99%の人が知らない睡眠の驚くべき真実と人生を変える科学的メカニズム
- review
人生が激変!『嫌われる勇気』岸見一郎・古賀史健著から学ぶ、アドラー心理学で手に入れる真の幸福と自己実現